clolsonus
Well-known member
I had a nugget of an idea tonight and was just wondering if something like this already exists. Background ... every time on a FT video they launch into some crazy new project, the say to start with some existing wing which is probably smart. But let's say I'm not one to follow advice, and I wanted something of a particular dimension (probably bigger than the average FT design) and wanted to figure out the sizes of things so it folded together right?
So I sat down and started puzzling through a python program where you would input the desired chord (let's say in mm) and it would spit out the dimensions you needed. It would kinda look like this (just a one evening hack, so very basic)
I could compute the lengths of the labeled edges (pad a little extra to make it around the leading edge radius) and would have enough to get out my ruler and start marking up a piece of foamboard. I could take it further and probably generate svg outlines given a wing span for the full cut file or at least a preview ...
Would this be stupid overkill? Is there something already far better? Is it just as easy to grab an existing wing design like FT recommends? Looking for thoughts/feedback if this would be useful or a waste of time? And I have plenty of other things to do so if it's a waste of time, be honest with me, I can take it!
Thanks!
Curt.
P.S. here's my quick hack python code.
So I sat down and started puzzling through a python program where you would input the desired chord (let's say in mm) and it would spit out the dimensions you needed. It would kinda look like this (just a one evening hack, so very basic)
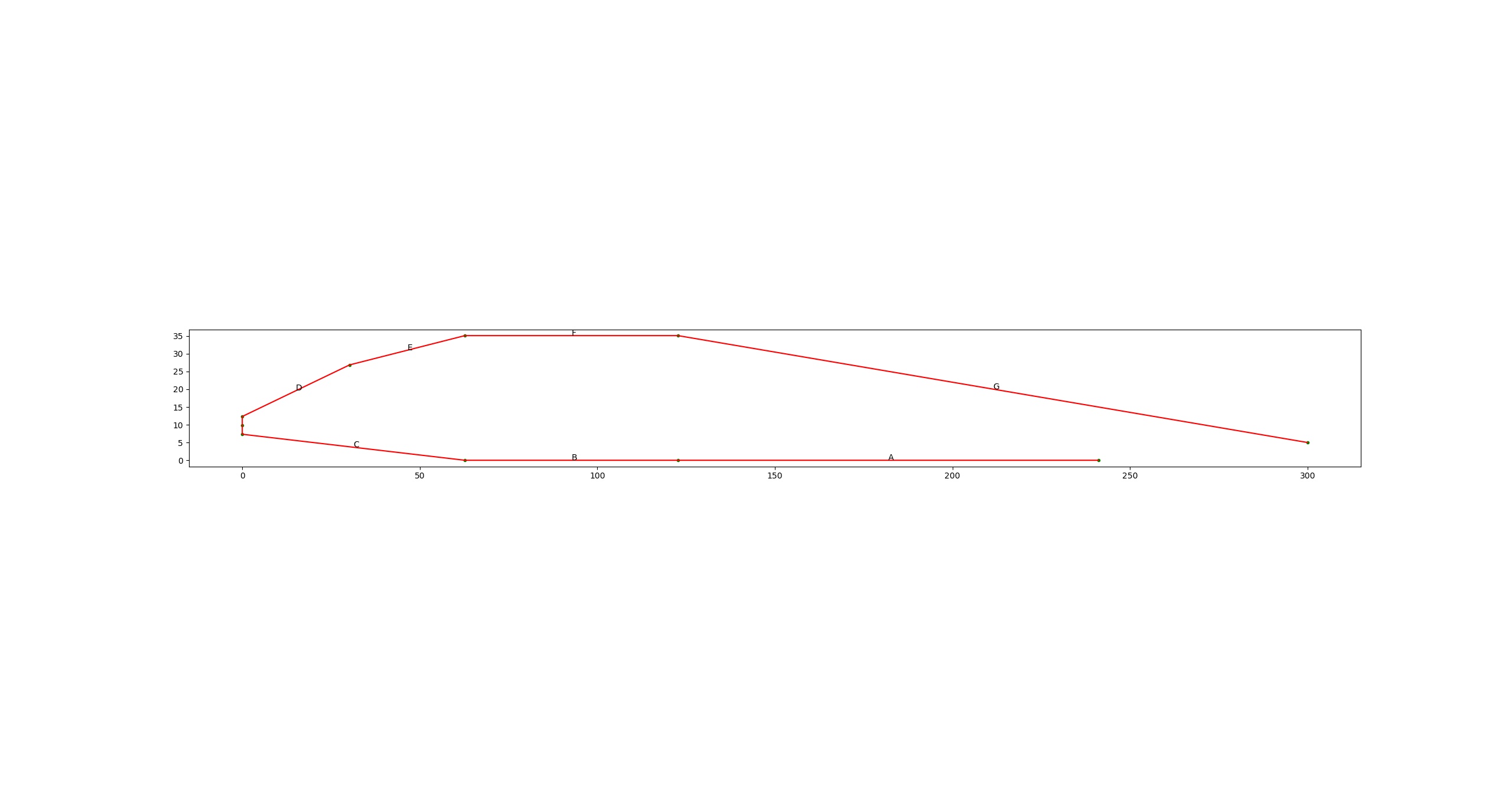
I could compute the lengths of the labeled edges (pad a little extra to make it around the leading edge radius) and would have enough to get out my ruler and start marking up a piece of foamboard. I could take it further and probably generate svg outlines given a wing span for the full cut file or at least a preview ...
Would this be stupid overkill? Is there something already far better? Is it just as easy to grab an existing wing design like FT recommends? Looking for thoughts/feedback if this would be useful or a waste of time? And I have plenty of other things to do so if it's a waste of time, be honest with me, I can take it!
Thanks!
Curt.
P.S. here's my quick hack python code.
Code:
#!/usr/bin/env python3
# this is a quick test to compute dimensions for a flight test style
# folded foam board wing ... loosely based on the clarky airfoil sorta
# kinda
import math
import matplotlib.pyplot as plt
import numpy as np
# units: let's do mm
r2d = 180 / math.pi
material_mm = 5 # mm
# clarky numbers
max_thickness_perc = 0.117 # vertically proportional to chord
max_point_perc = .309 # longitudinally proportional to chord
le_raise_perc = .28 # vertically proportional to max height
# basic proportions
spar_perc = 0.20 # longitudinally proportional to chord
aileron_perc = 0.23 # desired overhang for ailerons
# edit this to size wing
chord_mm = 300
print("wing chord mm: %.0f" % chord_mm)
# compute things
max_mm = chord_mm * max_thickness_perc
print("max thickness mm: %.0f" % max_mm)
le_height_mm = max_mm * le_raise_perc
spar_width_mm = chord_mm * spar_perc
spar_height_mm = max_mm - 2*material_mm
print("spar width mm: %.0f" % spar_width_mm)
print("spar height mm: %.0f" % spar_height_mm)
max_point_mm = chord_mm * max_point_perc
half_spar = spar_width_mm * 0.5
spar_start_mm = max_point_mm - half_spar
spar_end_mm = max_point_mm + half_spar
print("spar start mm: %.0f" % spar_start_mm)
print("spar end mm: %.0f" % spar_end_mm)
le_crease_mm = spar_start_mm * 0.5
print("leading edge crease mm: %.0f" % le_crease_mm)
ail_overhang_mm = chord_mm * aileron_perc
print("desired aileron overhang mm: %.0f" % ail_overhang_mm)
# do trigs
aft_dist = chord_mm - spar_end_mm
print(aft_dist)
h = max_mm - material_mm
aft_hyp = math.sqrt( (h*h) + (aft_dist*aft_dist) )
print(aft_hyp)
angle = math.asin(h/aft_hyp)
print("angle deg:", angle*r2d)
mat2 = material_mm*2
act_overhang_mm = mat2 / math.tan(angle)
print("actual overhang: %.0f" % act_overhang_mm)
# inner points
nose = [material_mm, le_height_mm]
bot_front_spar = [spar_start_mm, material_mm]
bot_rear_spar = [spar_end_mm, material_mm]
top_front_spar = [spar_start_mm, max_mm-material_mm]
top_rear_spar = [spar_end_mm, max_mm-material_mm]
bot_te = [chord_mm-act_overhang_mm, material_mm]
final_te = [chord_mm, 0]
inner = np.array([bot_te,
bot_rear_spar,
bot_front_spar,
nose,
top_front_spar,
top_rear_spar,
final_te
])
# compute outer points
nose_bot = [0, le_height_mm - material_mm*0.5]
nose_true = [0, le_height_mm]
nose_top = [0, le_height_mm + material_mm*0.5]
bot_front_spar = [spar_start_mm, 0]
bot_rear_spar = [spar_end_mm, 0]
top_front_spar = [spar_start_mm, max_mm]
top_rear_spar = [spar_end_mm, max_mm]
bot_te = [chord_mm-act_overhang_mm, 0]
final_te = [chord_mm, material_mm]
# dance
xdiff = spar_start_mm
ydiff = max_mm - nose_top[1]
len = math.sqrt(xdiff*xdiff + ydiff*ydiff)
base = [nose_top[0] + xdiff*0.5, nose_top[1] + ydiff*0.5]
xoff = -ydiff*0.05
yoff = xdiff*0.05
crease = [base[0]+xoff, base[1]+yoff]
outer = np.array([bot_te,
bot_rear_spar,
bot_front_spar,
nose_bot,
nose_true,
nose_top,
crease,
top_front_spar,
top_rear_spar,
final_te
])
le_pad = (material_mm*2*math.pi)/4 - material_mm
print("leading edge radius pad: %.1f" % le_pad)
def my_annotate(ax, text, p1, p2):
p = (np.array(p1) + np.array(p2))*0.5
pt = p.copy()
#if side == "top":
# pt[1] += material_mm
#else:
# pt[1] -= material_mm
ax.annotate(text, xy=p, xytext=pt)
#arrowprops=dict(facecolor='black', shrink=0.05),
#horizontalalignment='right', verticalalignment='top')
# plot
fig = plt.figure()
ax = fig.add_subplot()
x, y = outer.T
ax.scatter( x, y, marker=".", color="g" )
ax.plot( x, y, color="r" )
#x, y = inner.T
#ax.scatter( x, y, marker=".", color="g" )
#ax.plot( x, y, color="b")
my_annotate(ax, "A", outer[0], outer[1])
my_annotate(ax, "B", outer[1], outer[2])
my_annotate(ax, "C", outer[2], outer[3])
my_annotate(ax, "D", outer[5], outer[6])
my_annotate(ax, "E", outer[6], outer[7])
my_annotate(ax, "F", outer[7], outer[8])
my_annotate(ax, "G", outer[8], outer[9])
ax.set_aspect('equal')
plt.show()